2. Now, where did I put that value?
We've now seen that Elixir can handle words and numbers easily. But what else can it do? Well, it can remember things.
iex> sentence = "A really long and complex ⏎
sentence we'd rather not repeat."
"A really long and complex ⏎
sentence we'd rather not repeat."
iex> score = 2 / 5 * 100
40
iex> x = "not for multiplication"
"not for multiplication"
As long as we leave iex
running, Elixir will remember that sentence
is
"A really long and complex sentence we'd rather not repeat."
and score
is 40
,
and x
is "not for multiplication"
. At any point in time, we can ask it what
sentence
or score
or x
is and it will tell us:
iex> sentence
"A really long and complex sentence we'd rather not repeat."
iex> score
40
iex> x
"not for multiplication"
sentence
, score
and x
here are variables, and they're given that
particular name because the thing that the computer remembers with that name of sentence
or
score
or x
can vary. Remember when we talked about multiplying numbers and we saw
that we couldn't use x
to multiply? The reason for this is because Elixir would read x
as
a variable, not as a suggestion to multiply the numbers on either side. So we will continue using *
instead.
We can tell the computer to replace its definition of a variable with another one. This is often referred to as
re-assignment. Let's replace (or re-assign) the sentence
variable's definition:
iex> sentence = "An even longer and significantly more complex sentence ⏎
that we might be ok with repeating, if the mood takes us."
"An even longer and significantly more complex sentence ⏎
that we might be ok with repeating, if the mood takes us."
Then when we ask the computer what sentence
is, it will have forgotten the old sentence
and will only know the new one:
iex> sentence
"An even longer and significantly more complex sentence ⏎
that we might be ok with repeating, if the mood takes us."
Now the masses are looking chirpier. Some are even smiling! Isn't that wonderful? Let's create another variable
called place
:
iex> place = "World"
"World"
The computer will now remember that place
is "World"
. "But what's the use of just
setting a variable like this? Why make the computer remember at all?", Isodora (the spokesperson) says.
"Spokesperson, thanks", says Isodora, breaking the 4th wall again. Okay, Isodora (the spokesperson) said those
things. By the way, we have since learned the spokeswomanperson's name is Isodora, and so we've done some
variable creation of our own in our own brain: spokesperson = "Isodora"
. Woah. "Just call me Izzy",
says IsodoraIzzy. Ok, we will -- making our brains reassign the spokesperson
variable:
spokesperson = "Izzy"
.
Ok, Izzy's right. We should do something meaningful with this variable. Ok, Izzy, how about this? [cracks knuckles]
iex> "Hello, #{place}!"
"Hello, World!"
The masses go nuts. It's pandemonium! Then after a few shushing motions with my hands (which are completely ineffectual) and a quick "OI!" from Izzy, they're (mostly) quiet again.
"What just happened?", asks Izzy? But you, Dear Reader, had asked that question already. Izzy didn't hear because of the crowd and the sheer, unbounded pandemonium.
What on earth are those funky characters around place
? You can think of them as a placeholder.
Nerdier-types would call it interpolation. It tells the computer that we want to put the place
variable riiiiight here, right after Hello
and the space, and right before !
.
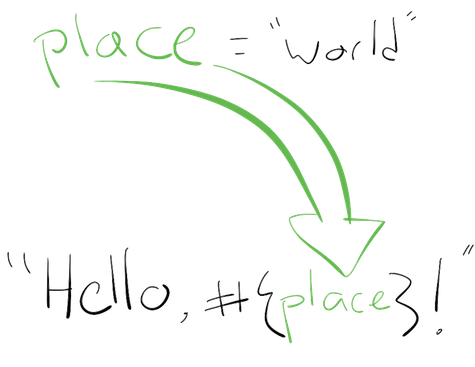
The computer takes the sentence that we give it, realizes that there's that funky #{}
placeholder
indicator, and it remembers what place
was and puts it right there. Pretty nifty, eh?
Exercises
- If we store the number of seconds in a day using this code:
seconds = 86400
, calculate using that variable how many hours there are in 30 days. - Create a variable called
name
, store a string in it and place the value of that variable in another string. - The line
5 / "four"
shows an error. Think about why this error might happen.