13. Finding more functions
We've now seen a small taste of a variety of functions that Elixir provides us. In this chapter, we're going to look at some new functions that will help us. These functions can show us documentation about other functions, tell us about variables within our code and more. We'll also see where we can go to find out more information
iex helper functions
The iex
prompt lets us run Elixir code with ease and to also see the output of that code. We've seen
plenty of examples of this throughout the last twelve chapters. But the iex
prompt has at least two
more tricks up its sleeves.
The h helper
The first of these tricks is the h
helper. This helper's name is short for "help", and it will
display documentation on whatever you wish. For instance, if you wanted to bring up the documentation for
Map.get/2
, you can do that by running this command inside of iex
:
iex> h Map.get/2
When you run this, you'll see this output:
def get(map, key, default \\ nil)
Gets the value for a specific key in map.
If key is present in map with value value, then value is returned. Otherwise,
default is returned (which is nil unless specified otherwise).
## Examples
iex> Map.get(%{}, :a)
nil
iex> Map.get(%{a: 1}, :a)
1
iex> Map.get(%{a: 1}, :b)
nil
iex> Map.get(%{a: 1}, :b, 3)
3
This output shows us the documentation that Elixir has for the Map.get/2
function. It even shows us
some examples of how to use this function, which is pretty great!
One thing we didn't learn back in Chapter 10 when we first learned about the Map.get/2
function is
that it can take a third argument too, a default argument. This argument will be returned if the key
we're trying to get isn't found, as we can see by the examples provided by the very helpful documentation.
"Hold on a minute!", Izzy pipes up. "If this function takes a third argument, then why is it called
Map.get/2
and not Map.get/3
?". Izzy makes a good point. The number at the end of
Map.get/2
signifies how many arguments a function takes. We learned this back in Chapter 9. What we
can see with this function is that there's a Map.get
variant that takes three arguments, and so there
is a Map.get/3
function, as well as the Map.get/2
.
We can look up any function's documentation that we've seen so far. Go on, try looking up the documentation for functions that we've seen in the past chapters. They're all documented here!
The arrow and v helpers
Two more little helpers that you should know about are the arrows on your keyboard, and the v
helper
provided by iex
. You might have been using the arrows already to move around in code. The up and down
arrows allow us to move through the historical input in our prompt, while the v
helper lets us pull
values out of particular lines in the output.
Let's say that we've been working in iex
and we've written this code:
iex(1)> %{name: "Izzy", age: "30ish", gender: "Female"}
iex(2)> %{name: "Ryan", age: "30ish", gender: "Male"}
Oh drat. We've just realised that wanted to assign these to variables, but forgot to. One way we can get that value again is by pressing the up-arrow on our keyboard. We'll then see this:
iex(1)> %{name: "Izzy", age: "30ish", gender: "Female"}
%{age: "30ish", gender: "Female", name: "Izzy"}
iex(2)> %{name: "Ryan", age: "30ish", gender: "Male"}
%{name: "Ryan", age: "30ish", gender: "Male"}
iex(3)> %{name: "Ryan", age: "30ish", gender: "Male"}
Our terminal helpfully brings back the last value when we push the up-arrow. But if we push the up-arrow again, look what happens:
iex(1)> %{name: "Izzy", age: "30ish", gender: "Female"}
%{age: "30ish", gender: "Female", name: "Izzy"}
iex(2)> %{name: "Ryan", age: "30ish", gender: "Male"}
%{name: "Ryan", age: "30ish", gender: "Male"}
iex(3)> %{age: "30ish", gender: "Female", name: "Izzy"}
We're now back to the first value again! We could then go to the start of this line (by pushing the left-arrow, or Ctrl+A) and assign it to a variable if we wished:
iex(3)> izzy = %{age: "30ish", gender: "Female", name: "Izzy"}
%{age: "30ish", gender: "Female", name: "Izzy"}
If we push the up-arrow again here, we'll get the same as line 3; the line we just had:
iex(4)> izzy = %{age: "30ish", gender: "Female", name: "Izzy"}
If we push it a second time, we'll get the same as line 2.
iex(4)> %{name: "Ryan", age: "30ish", gender: "Male"}
If we push the down-arrow, we'll go back to line 3.
iex(4)> izzy = %{age: "30ish", gender: "Female", name: "Izzy"}
This is how we can navigate up and down, or back and forward (depending your viewpoint) through the history of
what we've put into iex
.
Now about that v
helper. If we want, we can pick a value out of a particular line by using
v
. For instance, if we wanted the value from the 2nd line of our iex
prompt and then to
assign that to a variable called ryan
we can run this code:
iex(4)> ryan = v(2)
%{name: "Ryan", age: "30ish", gender: "Male"}
This code can be read as: find the 2nd value from the prompt, and assign it to a variable called
ryan
. As this v
helper is a function in Elixir, We can even leave off the brackets if we
wish to save us some typing:
iex(5)> ryan = v 2
%{name: "Ryan", age: "30ish", gender: "Male"}
Tab complete
One last iex
helper that I want to show you involves another key on your keyboard: the Tab
key.
In your iex
prompt, you can hit the Tab key to make it autocomplete what you type. For
instance, you can type this:
iex> String.rev
And then if you hit tab, iex
will autocomplete it into String.reverse
:
iex> String.reverse
You can do this with any module name or function you wish. Go on, give it a go with things like
En[TAB]
, and Enum.fil[TAB]
.
You might realise if you experiment enough that if iex
doesn't know what you want, then it will
provide some suggestions. For instance, if we type Enum.f
and hit tab, we'll see all the functions
from the Enum
module that start with f
:
fetch!/2 | fetch/2 | filter/2 |
find/2 | find/3 | find_index/2 |
flat_map_reduce/3 |
We've seen the Enum.find/2
and Enum.filter/2
functions back in Chapter 9, but as we can
see here Elixir provides a few more functions than the ones we've seen so far.
Keep on exploring here and seeing what other functions you can find. Remember that you can always use the
h
helper to bring up information about functions you don't know about yet. For instance, we could
bring up the documentation for Enum.find_index/2
by writing this:
iex> h Enum.find_index/2
The documentation that we would see is this:
def find_index(enumerable, fun)
@spec find_index(t(), (element() -> any())) :: non_neg_integer() | nil
Similar to find/3, but returns the index (zero-based) of the element instead of
the element itself.
## Examples
iex> Enum.find_index([2, 4, 6], fn x -> rem(x, 2) == 1 end)
nil
iex> Enum.find_index([2, 3, 4], fn x -> rem(x, 2) == 1 end)
1
There's also one more place where we can go to find documentation.
Elixir's documentation
As we've seen a few times now, we can bring up documentation in the iex
prompt with the
h
helper. There's another place where we can go to find the same documentation, as well as
documentation for every other function Elixir provides.
That place is https://elixir-lang.org/docs.html. When you visit that site, you'll see a list of documentation:
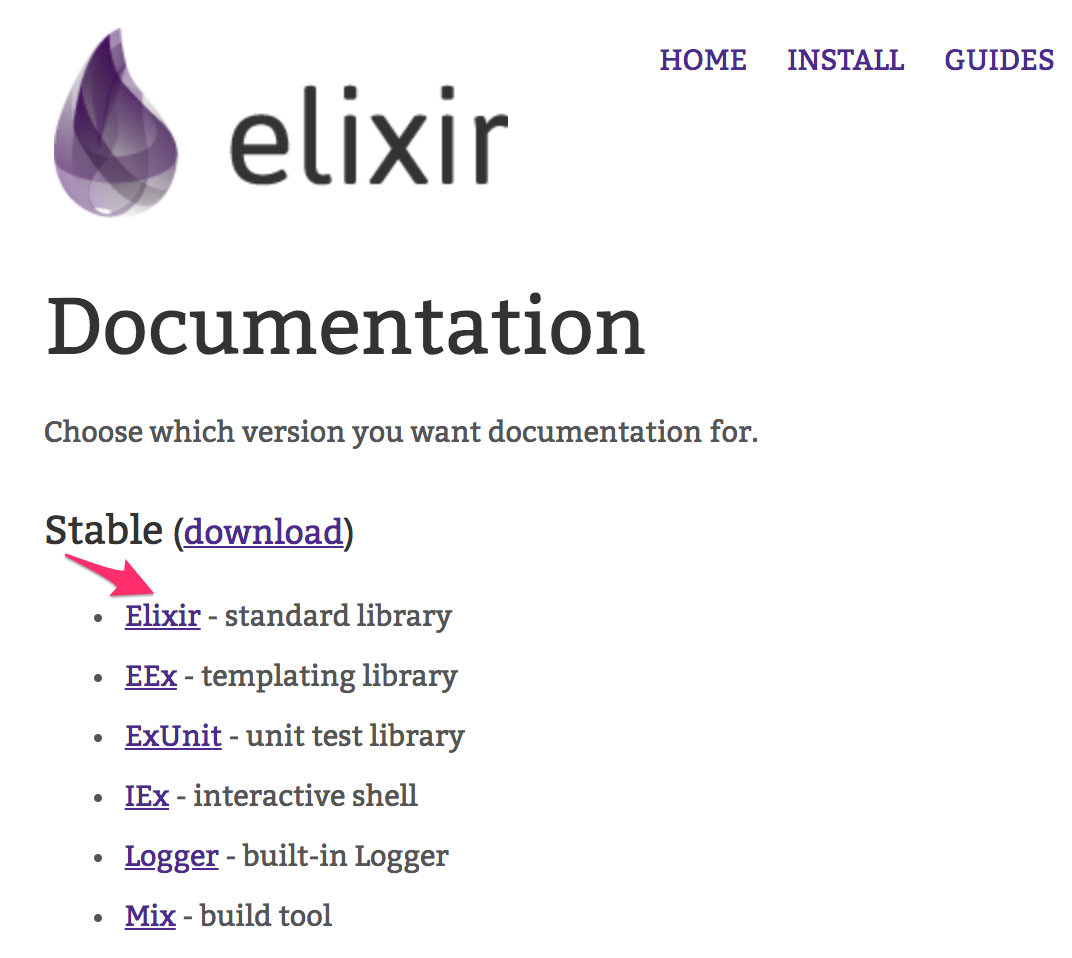
The "stable" name at the top indicates that the documentation listed underneath is the for the most recent stable release of Elixir, where "stable" meaning that it's safe for people like us to use, and won't have any nasty bugs in it.
We can see a list of documentation here for the different parts of Elixir. There's "Elixir", which is the documentation for all the functions we've been using so far. You can see documentation for other parts of Elixir too, including "IEx", which gets its own set of documentation. There's also "Mix" which we'll find out about in Chapter 14, and "ExUnit" that we'll find out about in Chapter 15.
If we click that "Elixir" link near the top, we'll be taken to the documentation site for Elixir's standard library. A "standard library" is a set of functions that programming languages provides, and this new page will show you Elixir's.
The default page is a little wordy:
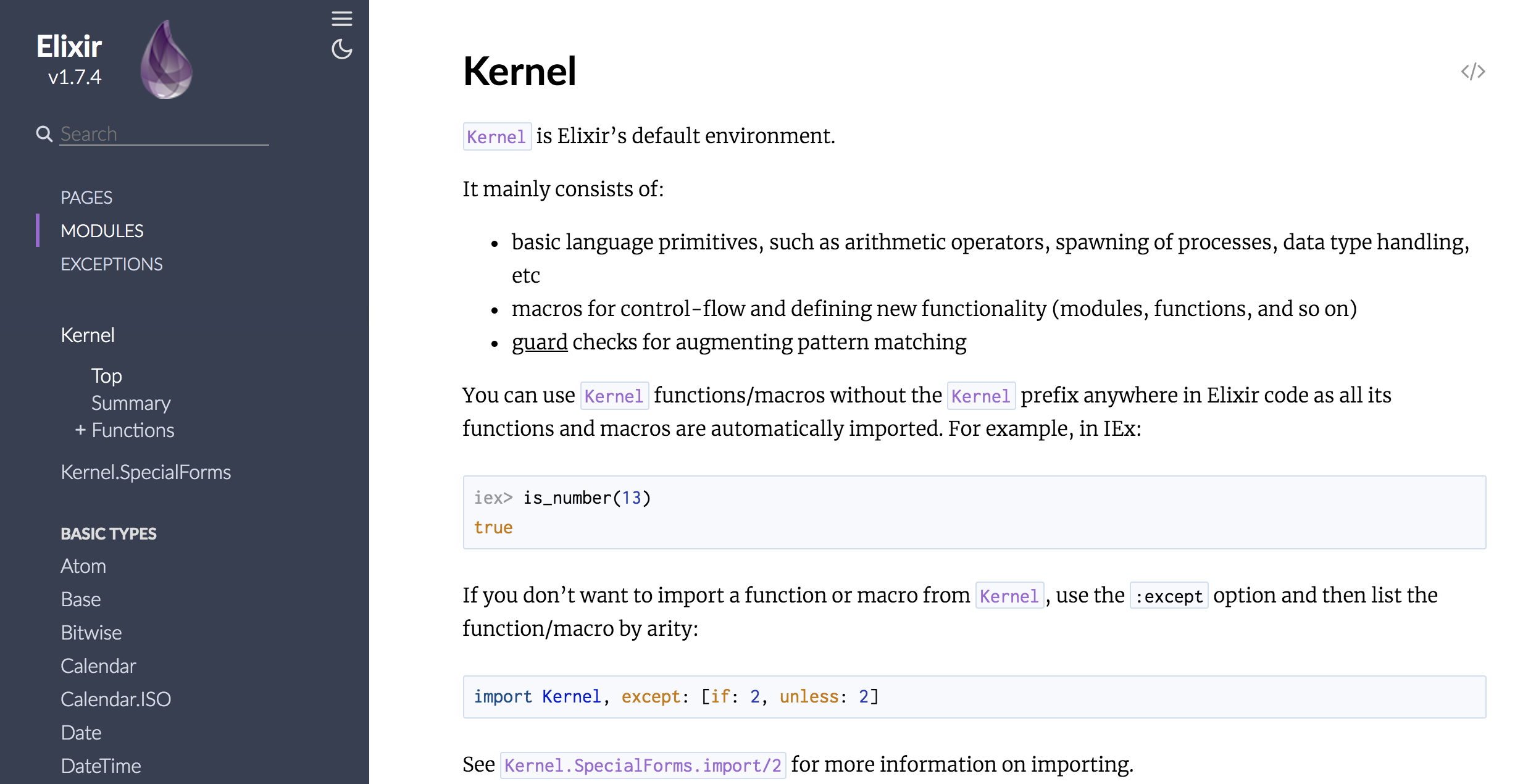
Let's not focus on all the words on the right-hand-side too much. What I want to show you here is the search bar over on the left.
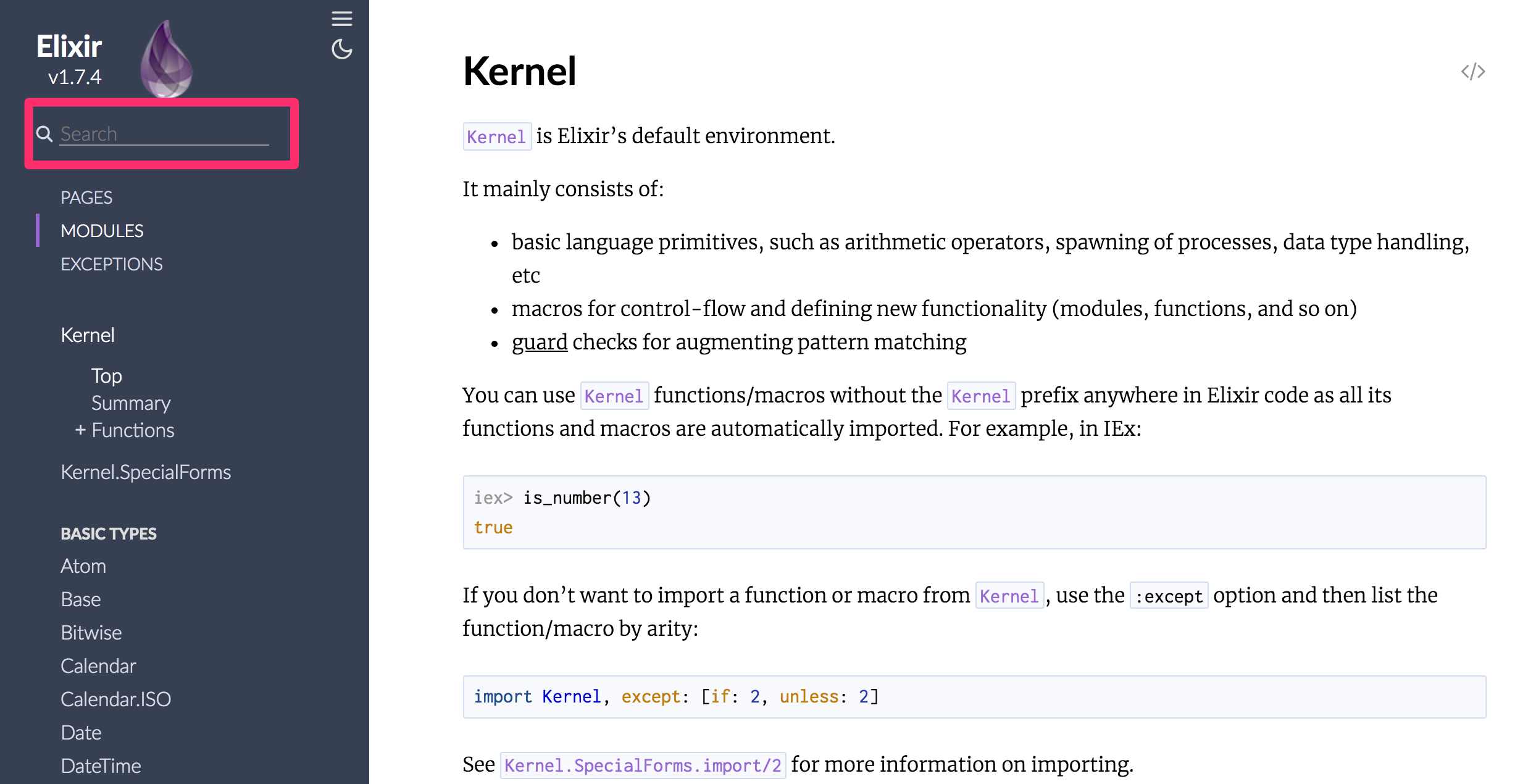
You can type any module or function name into this box to be taken right to its documentation. If we want to find
out about Enum.find
, we can search for that and then see this page:
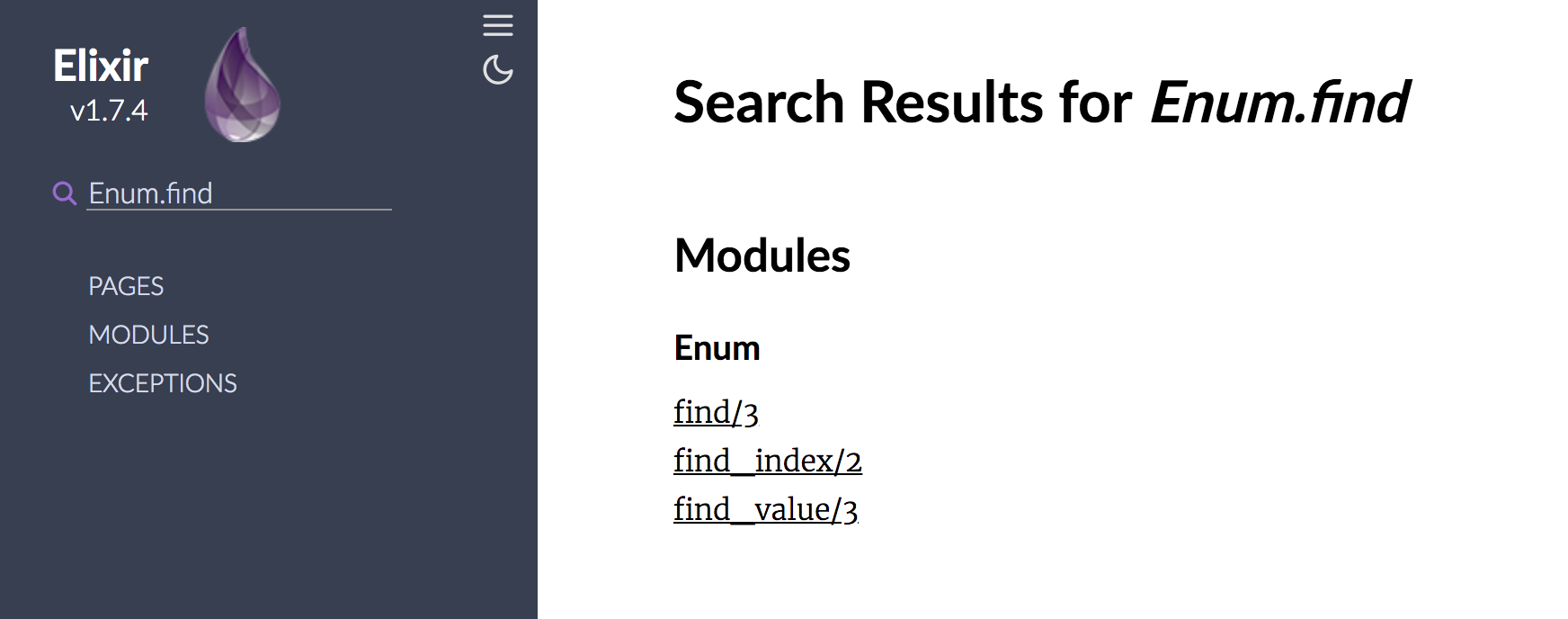
If we click on the find/3
function, we'll be taken right to its location in the docs: https://hexdocs.pm/elixir/Enum.html#find/3. The great
thing about this URL is that it's shareable, and so if you have friends that are learning Elixir too, you can give
them this URL to teach them about Enum.find/3
too.
Here's what we'll see on this page when we visit it.
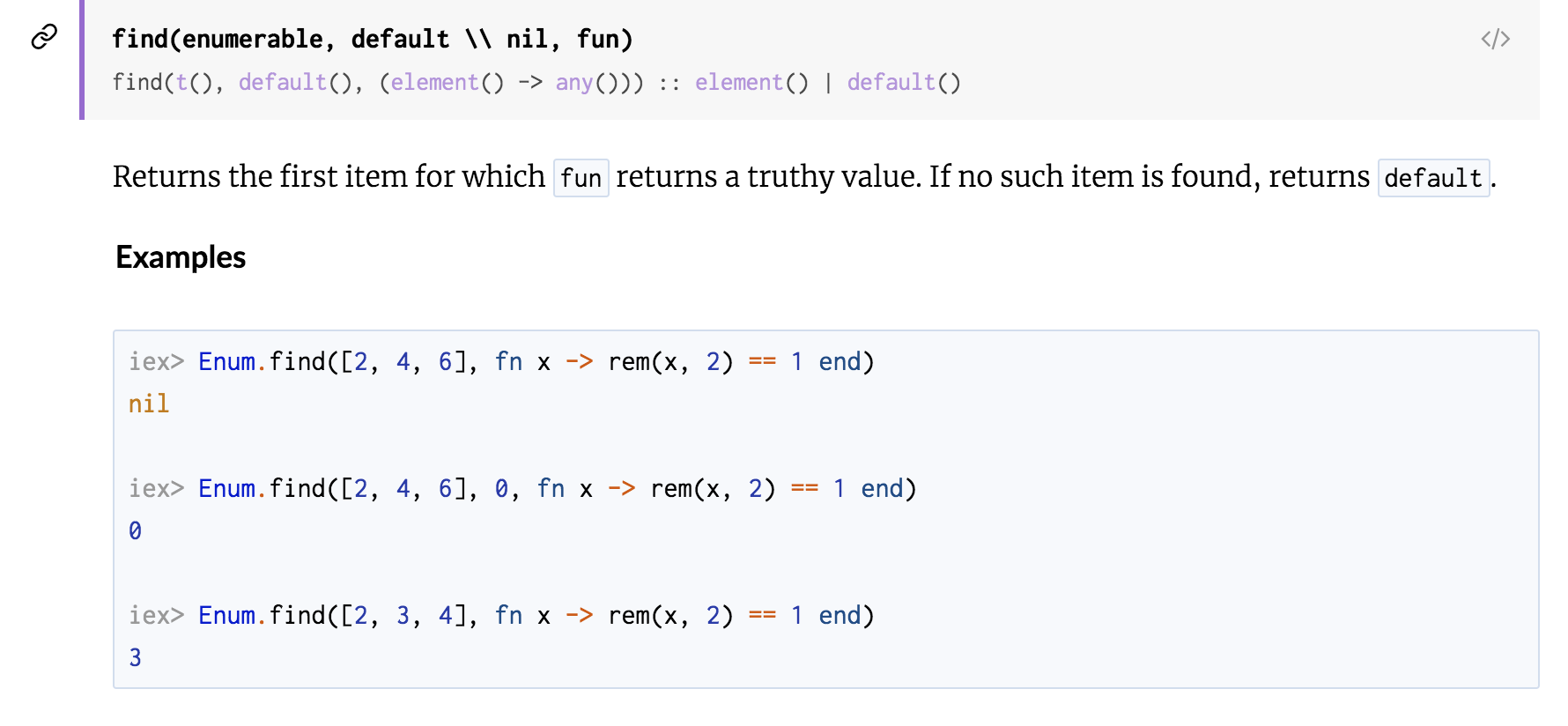
The great thing here is that we'll be on a page that has all the documentation that Elixir has for the
Enum
module. We can scroll up (or down!) the page to find out what other functions Elixir provides in
this module.
The other great thing about this is that the documentation here will match what we see when we use the
h
helper in the iex
prompt:
def find(enumerable, default \\ nil, fun)
@spec find(t(), default(), (element() -> any())) :: element() | default()
Returns the first item for which fun returns a truthy value. If no such item is
found, returns default.
## Examples
iex> Enum.find([2, 4, 6], fn x -> rem(x, 2) == 1 end)
nil
iex> Enum.find([2, 4, 6], 0, fn x -> rem(x, 2) == 1 end)
0
iex> Enum.find([2, 3, 4], fn x -> rem(x, 2) == 1 end)
3
So now we have two ways of looking at documentation for the functions that we're using within Elixir.
Elixir School
It would be remiss of me to talk about documentation and not to mention the other excellent source of documentation, written by the Elixir community for the Elixir community: Elixir School.
This site covers a lot of the same topics that we've covered -- and will cover in this book -- but in some particular cases it can provide different explanations for things. Now that you're this far into your Elixir journey, you should be able to read this and glean further knowledge from this site to bolster all the knowledge you've gained thus far.
Summary
In this chapter, we've looked at how we can be more productive by reading documentation and learning from that, as
well as new tricks for moving around in the iex
prompt.
We saw the h
helper, which brings up documentation about a particular module or function. We also saw
that we can press the up and down arrows on our keyboards to navigate through the history of what we've typed into
iex
Lastly, we saw that Elixir has online documentation that we can read too, and we saw that this documentation
matches exactly what we can see in our iex
prompt.
While we were looking at that documentation, we saw mentions of things called "Mix" and "ExUnit". These are two additional parts of Elixir's tooling that we will be looking at in the 4th and final part of this book: Chapters 14 and 15.